Implementing additional parameters to autocomplete in HubSpot
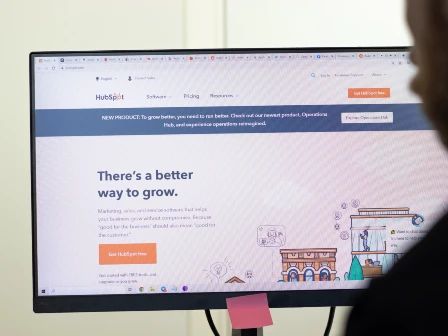
Most APIs are designed to go right in line with your code. As I showed in another article, sometimes you have to get your magical APIs to run outside of your fully controlled code. HubSpot is one of those places.
You can visit the other guide to figure out how to get started implementing an address autocomplete on your HubSpot form, or you can make that autocomplete work even better for you by setting additional parameters to that API javascript. We’ll discuss that here.
This supplemental guide explores the key customization options available through Smarty's US Address Autocomplete, from basic filtering to advanced geolocation preferences. You'll learn about:
- Basic configuration information
- Maximum number of results displayed
- How to include only certain cities, states, or ZIP Codes in displayed results
- How to exclude states from displayed results
- How to suggest certain cities, states, or ZIP Codes before others are suggested
- Preferring the user's geolocation or not
- Additional, less common parameters
- Conclusion
Let's dive into the practical implementations that will help you build more intelligent, user-friendly forms.
1. Basic configuration information
It would be wise not to mess with the majority of the HubSpot javascript provided in the main guide. However, there’s one section where you can add additional parameters to limit the results presented to the user. That section looks like this:
1 const sendLookupRequest = async (searchValue, selected = "") => {
2 const params = new URLSearchParams({
3 key: settings.embeddedKey,
4 search: searchValue,
5 source: MY_COOL_SMARTY_SOURCE,
6 selected
7 });
To find this block in your code, we suggest you do a "find on page" search and look for "const sendLookupRequest" as this is the only block that uses that line of code.
All of the following parameters will go after that line, between what we’ve called lines 5 and 6. You'll create a new line and paste it in, and it will be formatted the same as line 5.
It says "MY_COOL_SMARTY_SOURCE" because it’s referencing the parameter set at the top of that javascript block for ease of access. The following parameters won’t be set in those fun, easy-to-edit blocks up top but rather in the code block itself.
So let's talk about what that "source: "MY_COOL_SMARTY_SOURCE" (aka "postal") parameter is doing.
In the default HubSpot javascript code, we already included the parameter to determine the dataset the autocomplete tool looks at:
source: "MY_COOL_SMARTY_SOURCE",
By default, this parameter is set to "postal," meaning it’ll only show addresses in the USPS address database.
If you change that parameter to "all", then you'll also get Smarty's 20 million additional addresses that exist but aren't USPS addresses.
2. Maximum number of results displayed
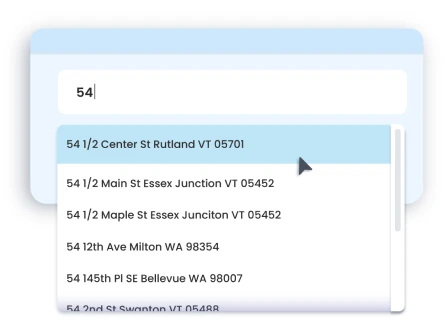
If you don't want the default 10 results to appear in your autocomplete suggestions, you'll need to add the following parameter:
max_results: #,
Of course, instead of the # sign, you'll put in the number of address suggestions you want displayed. The maximum number of suggestions is 10.
For example: max_results: 5,
So your block should now look like this:
1 const sendLookupRequest = async (searchValue, selected = "") => {
2 const params = new URLSearchParams({
3 key: settings.embeddedKey,
4 search: searchValue,
5 source: MY_COOL_SMARTY_SOURCE,
6 max_results: 5,
7 selected
8 });
After you update your page, your form will now suggest no more than 5 addresses at a time—or whatever number you landed on.
3. How to include only certain cities, states, or ZIP Codes in displayed results
Maybe you only do business in Georgia (GA) and Alabama (AL). It'd be a shame if your forms offer addresses in Utah or Washington, potentially offering service outside your area or making your Georgia customers look through all addresses before they get to their own.
To limit the addresses to a state or multiple states, add the following parameter:
include_only_states: "GA;AL",
In the GA;AL section, include the abbreviations for all the states you want included, separated by a semicolon and surrounded by quotation marks. If you only have one state, you don't need the semicolon.
Your block should now look like this:
1 const sendLookupRequest = async (searchValue, selected = "") => {
2 const params = new URLSearchParams({
3 key: settings.embeddedKey,
4 search: searchValue,
5 source: MY_COOL_SMARTY_SOURCE,
6 include_only_states: "GA;AL",
7 selected
8 });
Refresh your page. Now, your form won't suggest addresses outside of Georgia or Alabama.
You can also input "include_only" parameters for cities and ZIP Codes, as seen in the example below:
include_only_cities: "DENVER,AURORA,CO;OMAHA,NE",
include_only_zip_codes: "90210;06504",
Note that for cities, you'll need to also write out the state after the cities you include and separate each city/state set with a semicolon.
If you want to use any of the "include" or "exclude" parameters, it is recommended that you also add the "prefer_geolocation" parameter and ensure that it's set to "none". This is required for the include_only_zip_codes parameter to work.
4. How to exclude states from displayed results
If you do business in many states and it would be shorter to list the states you don't work in, this parameter is for you!
To exclude the states you don’t want to be shown in your autocomplete suggestions, add the following parameter:
exclude_states: "UT;CA;NV",
This example parameter will exclude Utah, California, and Nevada. You'll want to update yours to represent the states you want to exclude. Make sure that semicolons separate multiple states. A single state doesn’t require any semicolons.
Note: The "prefer_geolocation" parameter (line number 7 on this page) MUST be set to “none” if the user's current location is in a state specified in this parameter; otherwise, the customer will see addresses from their current location. This is because if you don't have the "prefer_gleolocation" parameter set, it defaults to "city" which will cause problems here, such as ignoring the exclusion rules so that it can include the IP city.
To avoid that, your code would need to look like this:
prefer_geolocation: "none",
So, within your block, it would look like this:
1 const sendLookupRequest = async (searchValue, selected = "") => {
2 const params = new URLSearchParams({
3 key: settings.embeddedKey,
4 search: searchValue,
5 source: MY_COOL_SMARTY_SOURCE,
6 exclude_states: "UT;CA;NV",
7 prefer_geolocation: "none",
8 selected
9 });
Refresh your page to ensure your form won't suggest any addresses within the states you've listed.
5. How to suggest certain cities, states, or ZIP Codes before others are suggested
Let's say you do most of your business in the ZIP Code 90210 (Ooooooh! Fancy.), but you still offer business outside of that ZIP Code. You can prioritize specific addresses before others by adding the following parameter:
prefer_zip_codes: "90210",
This example will show 90210 addresses first, but once someone types enough of their address that doesn't fit in that ZIP Code, it’ll suggest addresses outside of 90210.
If you want to provide additional ZIP Codes, you can as long as a semicolon separates them, like this:
prefer_zip_codes: "90210;90121;90212",
As we mentioned previously, this parameter also requires the "prefer_geolocation" parameter to be "none". That looks like this:
prefer_geolocation: "none",
Your code block looks like this:
1 const sendLookupRequest = async (searchValue, selected = "") => {
2 const params = new URLSearchParams({
3 key: settings.embeddedKey,
4 search: searchValue,
5 source: MY_COOL_SMARTY_SOURCE,
6 prefer_zip_codes: "90210",
7 prefer_geolocation: "none",
8 selected
9 });
Refresh your page. Your form will prioritize addresses within the ZIP Code 90210 or whatever you put there.
There are similar parameters for cities and states:
prefer_cities: "DENVER,AURORA,CO;OMAHA,NE",
prefer_states: "UT;ID;MT",
Note that for cities, you'll also need to include the state after the cities you list and separate each city/state set with a semicolon.
6. Preferring the user's geolocation or not
By default, the autocomplete tool will first read the user's IP address (IPv4 only) to determine the user's location and then automatically suggest addresses in that city and state. This is similar to the "prefer_cities" parameter, except it's dynamic to the user's location.
If you don't like that and want to go with the "exclude states" or the "prefer ZIP Codes" parameters, you'll need to set this parameter to "none". That looks like this:
prefer_geolocation: "none",
Or, in the code block:
1 const sendLookupRequest = async (searchValue, selected = "") => {
2 const params = new URLSearchParams({
3 key: settings.embeddedKey,
4 search: searchValue,
5 source: MY_COOL_SMARTY_SOURCE,
6 prefer_geolocation: "none",
7 selected
8 });
Refresh your page, and your form will stop prioritizing addresses at your user's geographic location.
Additional, less common parameters
You’ll find additional parameters and rules in the US Autocomplete Pro API documentation. We’ve included the most common examples above, and many love them as they are.
If you have additional questions, feel free to contact Smarty support. We can answer all your questions and get you up and running in no time.
Questions and conclusions
I showed all the examples above as individual parameters added to my code. Yes, it’s possible to have more than one of these parameters in your code. You'll just add them on their own lines with commas at the end of their lines. Some of them, like states and cities, become redundant together. But if you want to use the "max_results" parameter with the “prefer_geolocation” parameter, go ahead.
Check out the US Autocomplete API playground if you want to play with these parameters to see how they work and test them together without messing with your own code. Much like an actual playground, this is a safe place to try things without negative consequences. Unlike a real playground, it doesn't smell like feet.
Ready to get autocomplete in your HubSpot forms? Get more conversions today. We’ll even tell you the pricing upfront.